Crowd™


Join date : 31/01/2011 LostPoints : 10383 Thanks & Rep : 28 Posts : 229 Age : 33 Location : 6ret Warning Level : 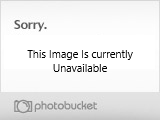
 | Subject: Encrypting and Decrypting files using DES Fri Aug 03, 2012 10:19 pm | |
| A few words about cryptography and DESCryptography is about hiding information, doesn't matter if it's plain text(ASCII) or binary data. In these days it is used for transmitting data safely, securing files, communication, etc. Key elements: source(file), key(file) and destination(file). To explain more about cryptography, I will use the therm, file, because in most cases the information is contained in files The source file is crypted with the help of an algorithm and a key, after the crypted file reached the destination it must be decrypted to be useful, with the same algorithm and key. Without knowing the key or the algorithm, the crypted file is useless, of course there are many methods and algorithms to break a crypted file, but that is not the purpose of this tutorial DES was one of the best algorithms for crypting in the world, but in the past years it was to many times broken, even the famous triple DES. Advantages: 56-bit key uses bit operations hardware implementation - fast, I mean god**** fast(originally designed for 1978 hardware) software implementation - relatively fast(but still fells like the wind blows your head of) Disadvantages: With now days machines it is breakable relatively fast, even with bruteforce. DES uses some tables, and hardcoded data, the algorithm its not clear, this is the reason why there where no enhancements, only the triple DES, but that is just using 3 times the encryption. [Only admins are allowed to see this link]Implementation in C#Fortunately .NET has many classes for data crypting, and it has DES to First, make a new C# project, a windows forms application, enter these using statements: - Code:
-
using System.IO; using System.Security.Cryptography; The interface of the application will look like this: [Only admins are allowed to see this image]Insert the openFileDialog and SaveFileDialog, objects, this will make file manipulation easier(It is usually a good practice, to not have file names hardcoded). Double click on the first button, or the one witch you whant to use for encryption. This method is the Encrypt Button event handler: - Code:
-
private void button1_Click(object sender, EventArgs e) { sKey = GenerateKey(); if (openFileDialog1.ShowDialog() == DialogResult.OK) { string source = openFileDialog1.FileName; saveFileDialog1.Filter = "des files |*.des"; if(saveFileDialog1.ShowDialog() == DialogResult.OK) { string destination = saveFileDialog1.FileName; EncryptFile(source, destination, sKey); } } MessageBox.Show("Succesfully Encrypted!"); } Here is the GenerateKey method, witch generates a key that will be used. - Code:
-
//function to generate a 64 bit key private string GenerateKey() { // Create an instance of Symetric Algorithm. Key and IV is generated automatically. DESCryptoServiceProvider desCrypto = (DESCryptoServiceProvider)DESCryptoServiceProvider.Create();
// Use the Automatically generated key for Encryption. return ASCIIEncoding.ASCII.GetString(desCrypto.Key);
} One more thing, the sKey string variable, must be a class variable, define it outside of any method, but inside your class, later I will add the full code. The EncryptFile, method: - Code:
-
private void EncryptFile(string source, string destination, string sKey) { FileStream fsInput = new FileStream(source, FileMode.Open, FileAccess.Read);
FileStream fsEncrypted = new FileStream(destination, FileMode.Create, FileAccess.Write);
DESCryptoServiceProvider DES = new DESCryptoServiceProvider(); DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey); DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey); ICryptoTransform desencrypt = DES.CreateEncryptor(); CryptoStream cryptostream = new CryptoStream(fsEncrypted, desencrypt, CryptoStreamMode.Write); byte[] bytearrayinput = new byte[fsInput.Length - 1]; fsInput.Read(bytearrayinput, 0, bytearrayinput.Length); cryptostream.Write(bytearrayinput, 0, bytearrayinput.Length); cryptostream.Close(); fsInput.Close(); fsEncrypted.Close(); } This method is the Decrypt Event handler: - Code:
-
private void button2_Click(object sender, EventArgs e) { openFileDialog1.Filter = "des files |*.des"; if (openFileDialog1.ShowDialog() == DialogResult.OK) { string source = openFileDialog1.FileName; if (saveFileDialog1.ShowDialog() == DialogResult.OK) { string destination = saveFileDialog1.FileName; DecryptFile(source, destination, sKey); } } MessageBox.Show("Succesfully Dencrypted!"); } And finally the DecryptFile method: - Code:
-
private void DecryptFile(string source, string destination, string sKey) { DESCryptoServiceProvider DES = new DESCryptoServiceProvider(); //A 64 bit key and IV is required for this provider. //Set secret key For DES algorithm. DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey); //Set initialization vector. DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey);
//Create a file stream to read the encrypted file back. FileStream fsread = new FileStream(source, FileMode.Open, FileAccess.Read); //Create a DES decryptor from the DES instance. ICryptoTransform desdecrypt = DES.CreateDecryptor(); //Create crypto stream set to read and do a //DES decryption transform on incoming bytes. CryptoStream cryptostreamDecr = new CryptoStream(fsread, desdecrypt, CryptoStreamMode.Read); //Print the contents of the decrypted file. StreamWriter fsDecrypted = new StreamWriter(destination); fsDecrypted.Write(new StreamReader(cryptostreamDecr).ReadToEnd()); fsDecrypted.Flush(); fsDecrypted.Close(); } This is my first tutorial, I hope you like it If you have some questions about the code and tutorial or criticism, feel free to post. Sorry for any writing or semantic mistakes or my english - Code:
-
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; using System.IO; using System.Security.Cryptography;
namespace DES { public partial class Form1 : Form { static string sKey; public Form1() { InitializeComponent(); } private void button1_Click(object sender, EventArgs e) { sKey = GenerateKey(); if (openFileDialog1.ShowDialog() == DialogResult.OK) { string source = openFileDialog1.FileName; saveFileDialog1.Filter = "des files |*.des"; if(saveFileDialog1.ShowDialog() == DialogResult.OK) { string destination = saveFileDialog1.FileName; EncryptFile(source, destination, sKey); } } MessageBox.Show("Succesfully Encrypted!"); }
private void EncryptFile(string source, string destination, string sKey) { FileStream fsInput = new FileStream(source, FileMode.Open, FileAccess.Read);
FileStream fsEncrypted = new FileStream(destination, FileMode.Create, FileAccess.Write);
DESCryptoServiceProvider DES = new DESCryptoServiceProvider(); DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey); DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey); ICryptoTransform desencrypt = DES.CreateEncryptor(); CryptoStream cryptostream = new CryptoStream(fsEncrypted, desencrypt, CryptoStreamMode.Write); byte[] bytearrayinput = new byte[fsInput.Length - 1]; fsInput.Read(bytearrayinput, 0, bytearrayinput.Length); cryptostream.Write(bytearrayinput, 0, bytearrayinput.Length); cryptostream.Close(); fsInput.Close(); fsEncrypted.Close();
}
//function to generate a 64 bit key private string GenerateKey() { // Create an instance of Symetric Algorithm. Key and IV is generated automatically. DESCryptoServiceProvider desCrypto = (DESCryptoServiceProvider)DESCryptoServiceProvider.Create();
// Use the Automatically generated key for Encryption. return ASCIIEncoding.ASCII.GetString(desCrypto.Key);
}
private void button2_Click(object sender, EventArgs e) { openFileDialog1.Filter = "des files |*.des"; if (openFileDialog1.ShowDialog() == DialogResult.OK) { string source = openFileDialog1.FileName; if (saveFileDialog1.ShowDialog() == DialogResult.OK) { string destination = saveFileDialog1.FileName; DecryptFile(source, destination, sKey); } } MessageBox.Show("Succesfully Dencrypted!");
}
private void DecryptFile(string source, string destination, string sKey) { DESCryptoServiceProvider DES = new DESCryptoServiceProvider(); //A 64 bit key and IV is required for this provider. //Set secret key For DES algorithm. DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey); //Set initialization vector. DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey);
//Create a file stream to read the encrypted file back. FileStream fsread = new FileStream(source, FileMode.Open, FileAccess.Read); //Create a DES decryptor from the DES instance. ICryptoTransform desdecrypt = DES.CreateDecryptor(); //Create crypto stream set to read and do a //DES decryption transform on incoming bytes. CryptoStream cryptostreamDecr = new CryptoStream(fsread, desdecrypt, CryptoStreamMode.Read); //Print the contents of the decrypted file. StreamWriter fsDecrypted = new StreamWriter(destination); fsDecrypted.Write(new StreamReader(cryptostreamDecr).ReadToEnd()); fsDecrypted.Flush(); fsDecrypted.Close();
} } } Enjoy ^^ Credit For me | |
|